In this article, let us discuss about passing data from Parent Component to Child Component using @Input() decorator
To send data from parent component, a component property in child component must be declared as Input property using @Input decorator
Step 1: Create the project, child compoent, parent component from Console using Angular CLI commands as below
C:\Users\Girish\angularprojs>ng new myAngFirst
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
CREATE myAngFirst/angular.json (3625 bytes)
CREATE myAngFirst/package.json (1286 bytes)
.................
C:\Users\Girish\angularprojs\myAngFirst>ng g c parent
CREATE src/app/parent/parent.component.html (21 bytes)
CREATE src/app/parent/parent.component.spec.ts (628 bytes)
CREATE src/app/parent/parent.component.ts (269 bytes)
CREATE src/app/parent/parent.component.css (0 bytes)
UPDATE src/app/app.module.ts (475 bytes)
..................
C:\Users\Girish\angularprojs\myAngFirst>ng g c child
CREATE src/app/child/child.component.html (20 bytes)
CREATE src/app/child/child.component.spec.ts (621 bytes)
CREATE src/app/child/child.component.ts (265 bytes)
CREATE src/app/child/child.component.css (0 bytes)
UPDATE src/app/app.module.ts (553 bytes)
Let us display courses information with properties code, course name, fee, student name in a table
Step 2: Inside the child component write the code like below (child.component.ts
). Here we have converted the Component Properties all, python and angular to Input properties by add @Input decorator to each property. Also import Input from ‘@angular/core’
import { Component, OnInit, Input } from '@angular/core';
@Component({
selector: 'app-child',
templateUrl: './child.component.html',
styleUrls: ['./child.component.css']
})
export class ChildComponent implements OnInit {
@Input() all: number;
@Input() python: number;
@Input() angular: number;
constructor() { }
ngOnInit() {
}
}
Now write the below code for the (child.component.html
)
<span class="radioClass">Show : </span>
<input name='options' type='radio' value="All">
<span class="radioClass">{{'All(' + all + ')'}}</span>
<input name="options" type="radio" value="Python">
<span class="radioClass">{{"Python(" + python + ")"}}</span>
<input name="options" type="radio" value="Angular">
<span class="radioClass">{{"Angular(" + angular + ")"}}</span>
Step 3: Inside the parent component write the code like below (parent.component.ts
). Here we have created courses object array inside constructor and created getTotalCourseCount(), getPythonCourseCount() and getAngularCourseCount(), to get the count of the courses.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-parent',
templateUrl: './parent.component.html',
styleUrls: ['./parent.component.css']
})
export class ParentComponent implements OnInit {
constructor() {
this.courses = [
{
coursecode: 'PY', coursename: 'Python', fee: 5500, student: 'Girish'
},
{
coursecode: 'NG', coursename: 'Angular', fee: 3000, student: 'Santosh'
},
{
coursecode: 'NG', coursename: 'Angular', fee: 3000, student: 'Sanjay'
},
{
coursecode: 'PY', coursename: 'Python', fee: 3000, student: 'Ramesh'
},
{
coursecode: 'PY', coursename: 'Python', fee: 5500, student: 'Ganesh'
},
{
coursecode: 'PY', coursename: 'Python', fee: 5500, student: 'Govind'
}, {
coursecode: 'PY', coursename: 'Python', fee: 5500, student: 'Govind'
},
];
}
getTotalCourseCount(): number {
return this.courses.length;
}
getPythonCourseCount(): number {
return this.courses.filter(e => e.coursename === 'Python').length;
}
getAngularCourseCount(): number {
return this.courses.filter(e => e.coursename === 'Angular').length;
}
ngOnInit() {
}
}
Now write the below code for the (parent.component.html
) . Here we have binded the methods of Parent Component to Child Component to fetch and display the course count.
<app-child [all]="getTotalCourseCount()"
[python]="getPythonCourseCount()"
[angular]="getAngularCourseCount()">
</app-child>
<br /><br />
<table>
<thead>
<tr>
<th>Code</th>
<th>Name</th>
<th>Fee</th>
<th>Student</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let course of courses;">
<td>{{course.coursecode}}</td>
<td>{{course.coursename}}</td>
<td>{{course.fee}}</td>
<td>{{course.student}}</td>
</tr>
<tr *ngIf="!courses || courses.length==0">
<td colspan="5">
No courses to display
</td>
</tr>
</tbody>
</table>
At this point, save all the changes and run the application and you can see the correct count of courses next to each radio button.
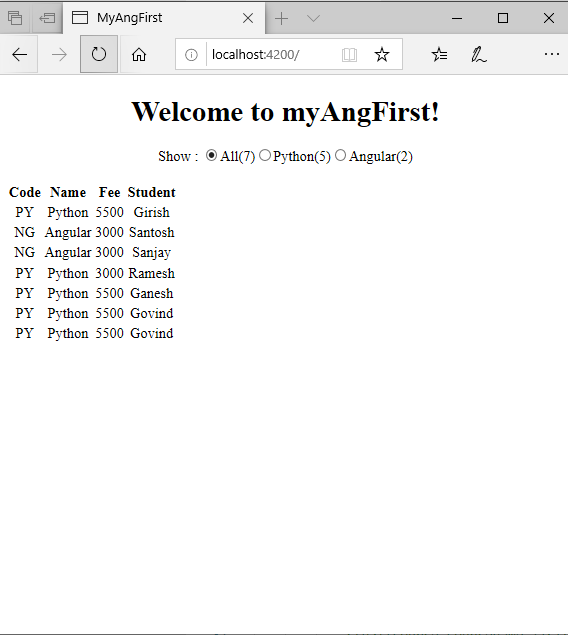
Now, let’s add the following new course object to the courses array in ParentComponent and Save changes and reload the web page and notice that All count and Angular count is increased by 1 as expected.
{
coursecode: 'PY', coursename: 'Angular', fee: 5500, student: 'Saravana'
}
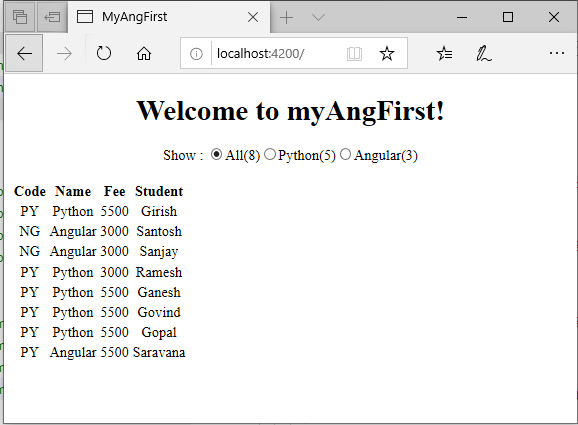
References
- https://angular.io/guide/template-syntax#input-and-output-properties
- https://angular.io/api/core/Input
In the next article, we will discuss about sending user events from Child Component to Parent Component using @Output() decorator, @EventEmitter and ng-container directive.
Happy Learning!