- The Spring Framework is an Open Source application framework and inversion of control container for the Java platform.
- Spring Framework allows allows Java developers to build simple, reliable, and scalable enterprise applications.
- Latest version of Spring Framework is 5.2.0.RELEASE relased on September 30, 2019
- Official website is
https://spring.io/
Step 1: Download Spring Tools 4 for Eclipse from https://spring.io/tools
Spring Tools 4 is the next generation of Spring tooling for your favorite coding environment. Largely rebuilt from scratch, it provides world-class support for developing Spring-based enterprise applications
Step 2: Create Maven Project from STS File > New > Maven Project as show below
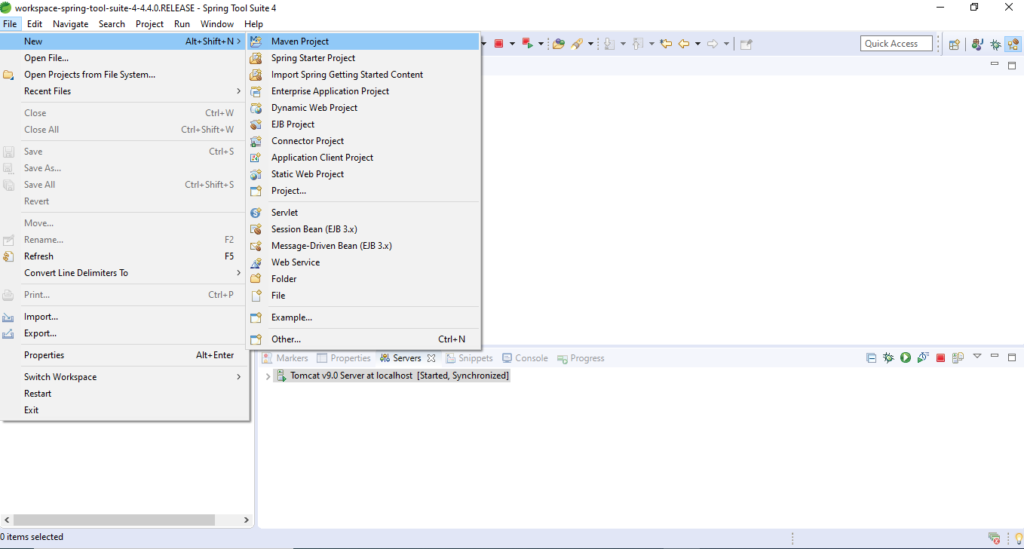
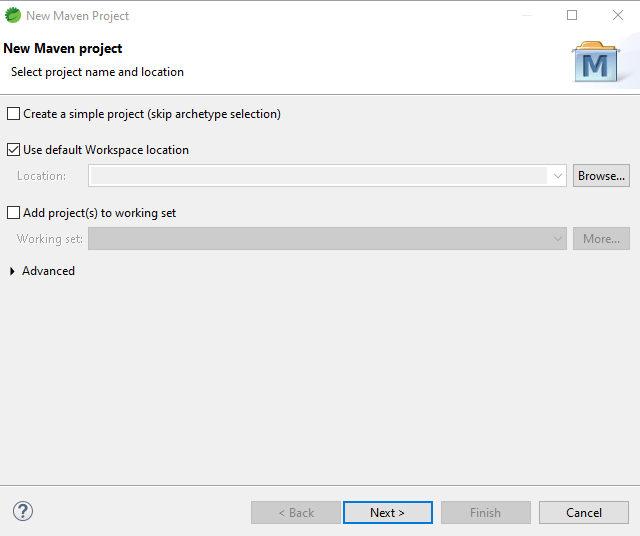
Select maven-archetype-webapp
Artifact Id
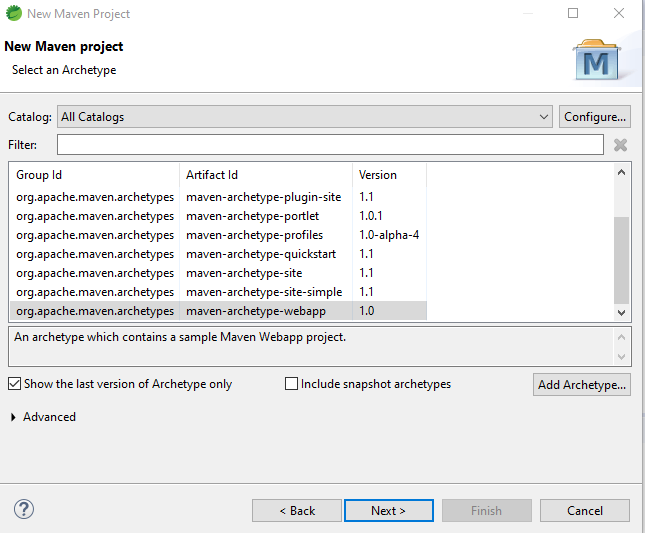
Now fill the below details
- Group id : It uniquely identifies your project, like com.companyname
- Artifact id : It is name of jar or war without version, like project name
- Version : Version is used for version control for artifact id.
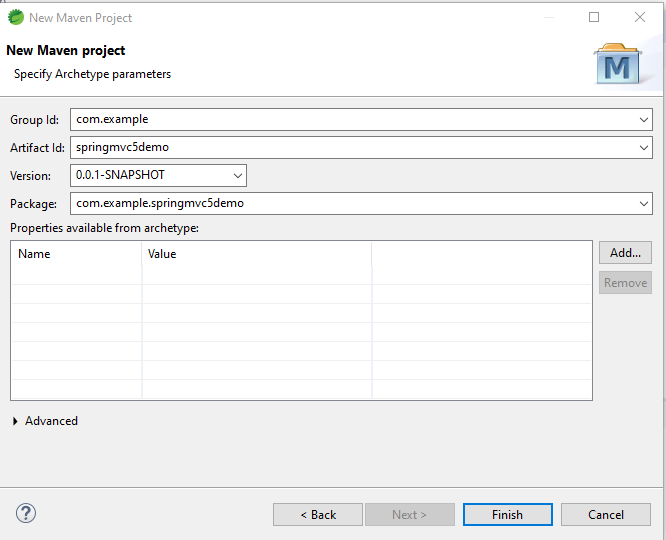
Once the project is created, the project structure looks like as shown below.
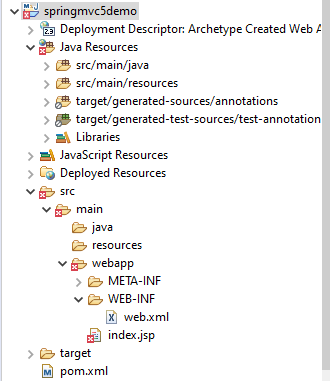
You may get an error showing “javax.servlet.http.HttpServlet” was found in java build path” in index.jsp. To solve this issue you need to set target run time as Apache Tomcat , right click on project -> properties -> target runtimes
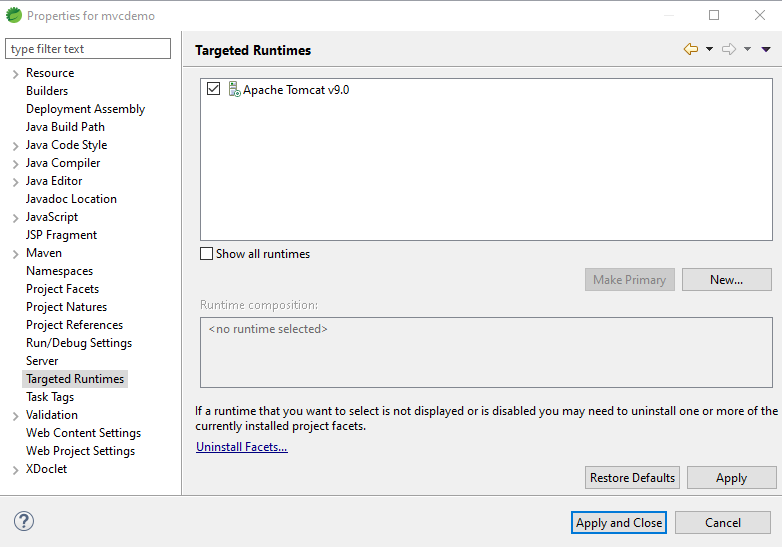
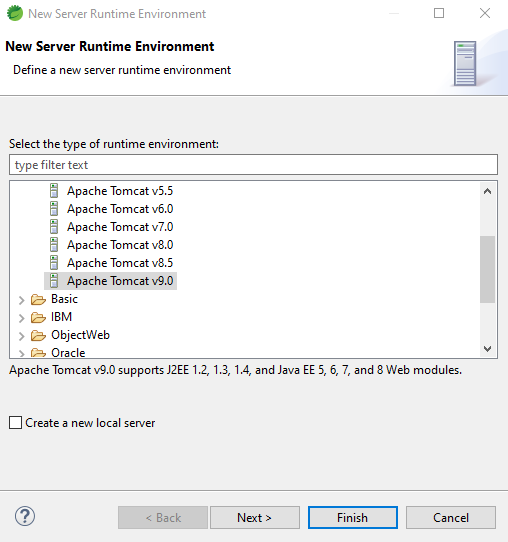
Download Tomcat Server, unzip and keep in one folder
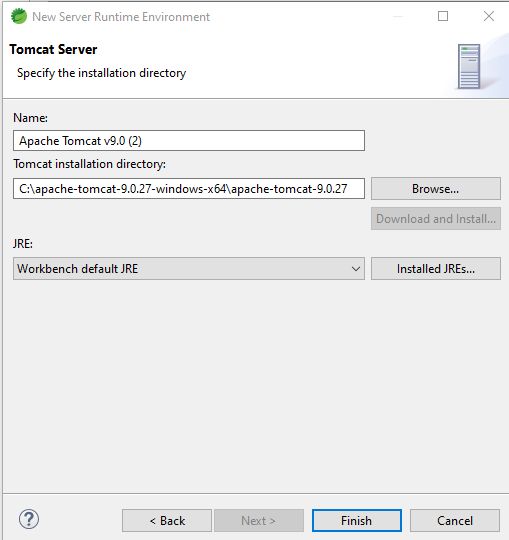
Now add Spring Dependency in pom.xml
file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>springmvc5demo</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>springmvc5demo Maven Webapp</name>
<url>http://maven.apache.org</url>
<properties>
<failOnMissingWebXml>false</failOnMissingWebXml>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.1.0.RELEASE</version>
</dependency>
<!-- JSTL Dependency -->
<dependency>
<groupId>javax.servlet.jsp.jstl</groupId>
<artifactId>javax.servlet.jsp.jstl-api</artifactId>
<version>1.2.1</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<!-- Servlet Dependency -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- JSP Dependency -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.3.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>springmvc5demo</finalName>
</build>
</project>
Now create packages under src/main/java
as show in the below screenshot
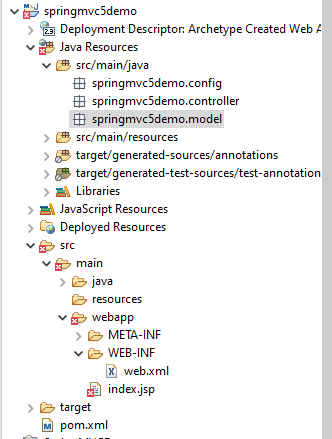
Now Create AppConfig.java, DispatcherServletInitializer.java under springmvc5demo.config package.
Create Course.java under springmvc5demo.model
Create CourseController.java under springmvc5demo.controller
AppConfig.java
package springmvc5demo.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
import org.springframework.web.servlet.view.JstlView;
@Configuration
@EnableWebMvc
@ComponentScan(basePackages = {
"springmvc5demo.config","springmvc5demo.controller", "springmvc5demo.model"
})
public class AppConfig {
@Bean
public InternalResourceViewResolver resolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setViewClass(JstlView.class);
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
}
DispatcherServletInitializer.java
package springmvc5demo.config;
import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer;
public class DispatcherServletInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class <?> [] getRootConfigClasses() {
// TODO Auto-generated method stub
return null;
}
@Override
protected Class <?> [] getServletConfigClasses() {
return new Class[] {
AppConfig.class
};
}
@Override
protected String[] getServletMappings() {
return new String[] {
"/"
};
}
}
Course.java
package springmvc5demo.model;
public class Course {
private String courseName;
private String courseInstructor;
public String getCourseName() {
return courseName;
}
public void setCourseName(String courseName) {
this.courseName = courseName;
}
public String getCourseInstructor() {
return courseInstructor;
}
public void setCourseInstructor(String courseInstructor) {
this.courseInstructor = courseInstructor;
}
}
CourseController.java
package springmvc5demo.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import springmvc5demo.model.Course;
@Controller
public class CourseController {
@RequestMapping("/hello")
public String handler(Model model) {
Course cou = new Course();
cou.setCourseName("Spring Framework");
cou.setCourseInstructor("Girish");
model.addAttribute("hello", cou);
return "hello";
}
}
- First Request goes to dispatcherServlet and it redirects to controller class.
- Here @Controller depicts that this is our controller class.
- @RequestMapping is used to map incoming http request to handler method
- handler() method of MyController.java will handle GET request from dispatcher.
Create an hello.jsp file under src/main/webapp/WEB-INF/views folder and write the below code
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head><%@ page isELIgnored="false" %>
<meta charset="ISO-8859-1">
<title>Spring 5 MVC - Hello World Example</title>
</head>
<body>
<h2>${hello.courseName}</h2>
<h1>${hello.courseInstructor}</h1>
</body>
</html>
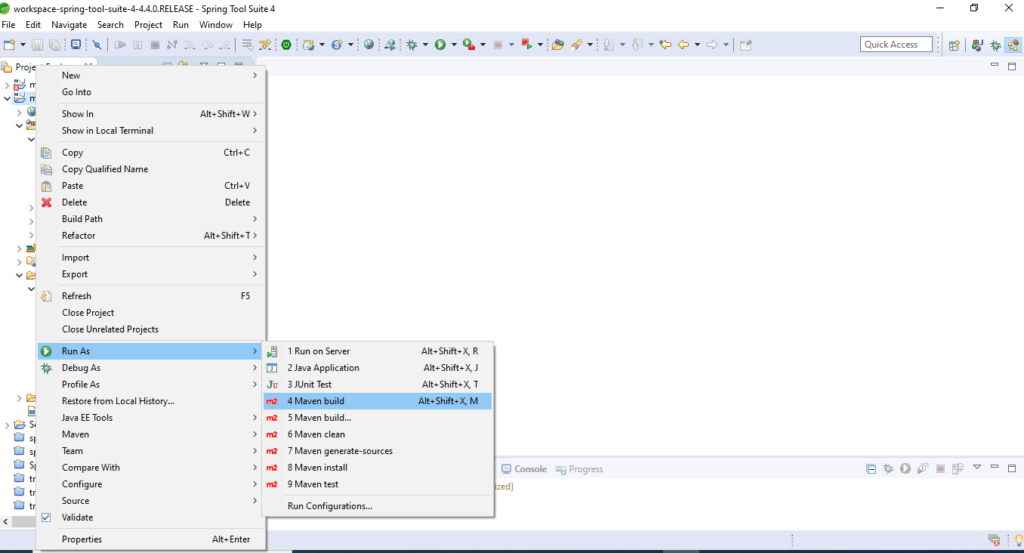
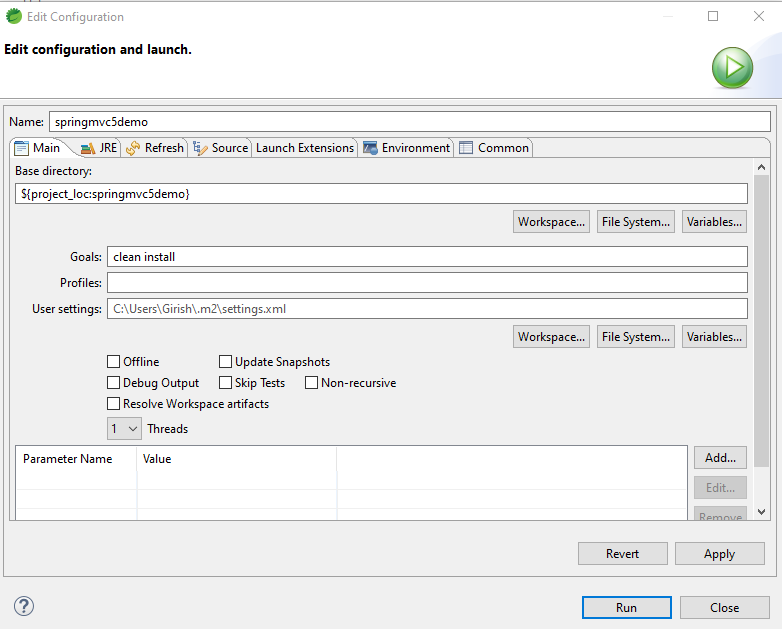
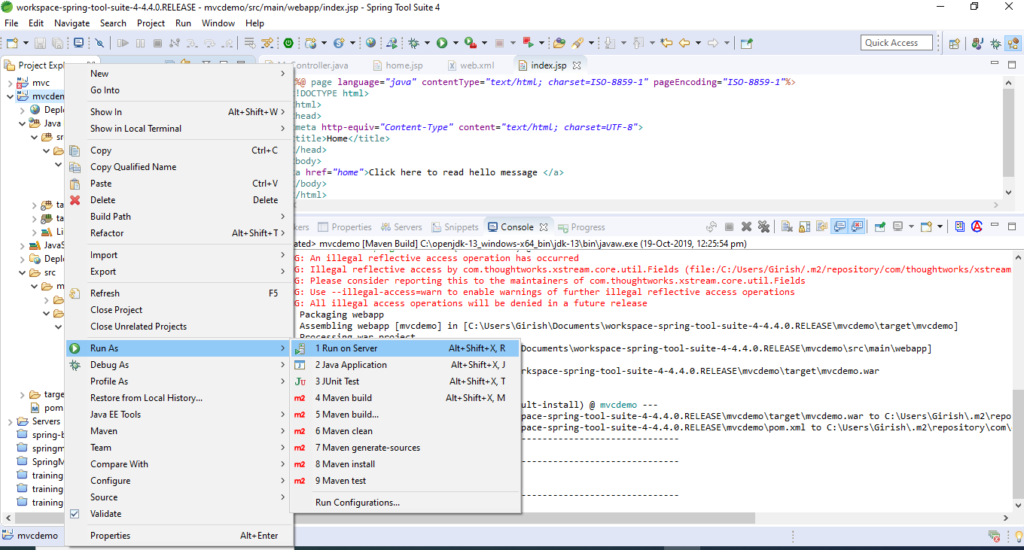
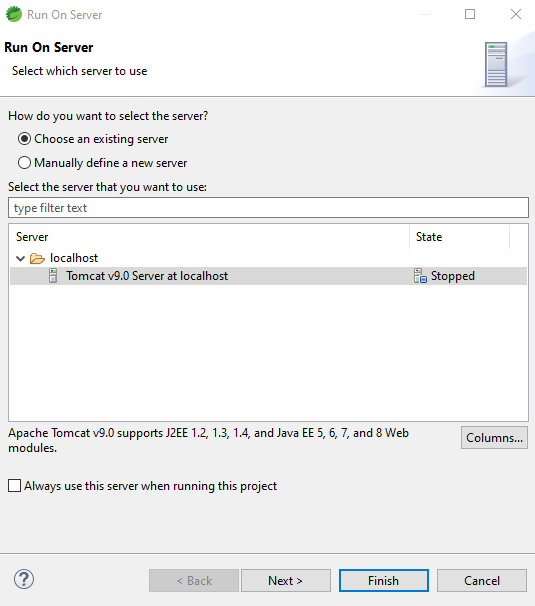
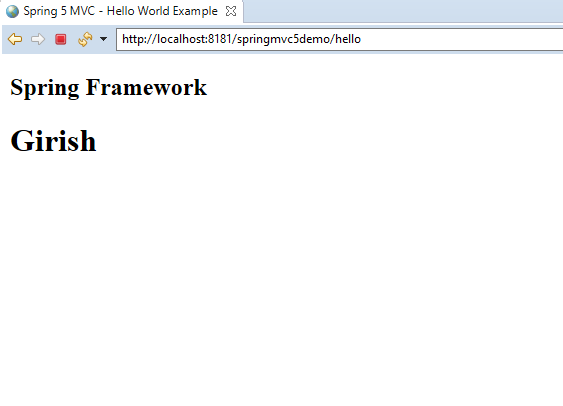
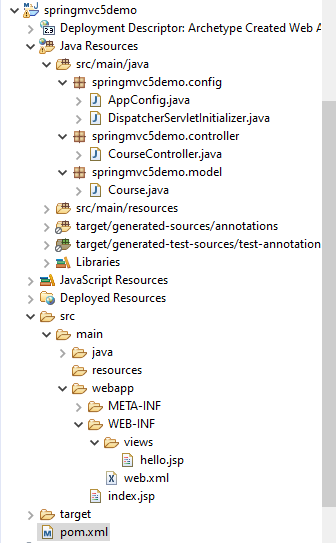
In this article we used Java-based configuration by configuring DispatcherServlet and spring beans configuration.
Tools and Technologies used in this example
- Spring MVC – 5.1.0 RELEASE
- JDK – 13
- Maven – 3.6.1
- Apache Tomcat – 9.0
- IDE – STS 4
- JSTL – 1.2.1
References
- https://docs.spring.io/spring/docs/5.2.0.RELEASE/spring-framework-reference/
Learn more about Spring Framework in the upcoming blog articles.
Happy Learning!