- TypeScript is a typed superset of JavaScript that compiles to plain JavaScript.
- It offers classes, modules, and interfaces to help you build robust components.
- TypeScript compiles to clean, simple JavaScript code which runs on any browser, in Node.js, or in any JavaScript engine that supports ECMAScript 3 (or newer).
Step 1: Install Node.js and NPM
Step 2: Install TypeScript globally using NPM from windows console as below
C:\Users\Girish>npm install -g typescript
To test the TypeScript version
C:\Users\Girish>tsc --version
Version 3.6.3
Step 3: Create helloworld.ts
file as below
let message: string = 'Hello World';
console.log(message);
Step 4: Now compile the helloworld.ts
file as below
C:\Users\Girish\myTSProj>tsc helloworld.ts
Once the helloworld.ts file is compiles you can see helloworld.js
file generated. You can use this run via node or from html file
Step 5: Run from Node and you can see the output of the code in the console window
C:\Users\Girish\myTSProj>node helloworld.js
Hello World
Step 6: Let us do some modifications to helloworld.ts
file and create new helloworld.html
file as below
helloworld.ts
file
let message: string = 'Hello World';
//console.log(message);
//document.body.innerHTML = '<h1>' + message + '</h1>';
document.getElementById("demo").innerHTML = message ;
helloworld.html
file
<!DOCTYPE html>
<html>
<head><title>TypeScript Hello World</title>
</head>
<body>
<div id="demo"></div>
<script src="helloworld.js"></script>
</body>
</html>
Now open the helloworld.html
file in the browser and you can see like this
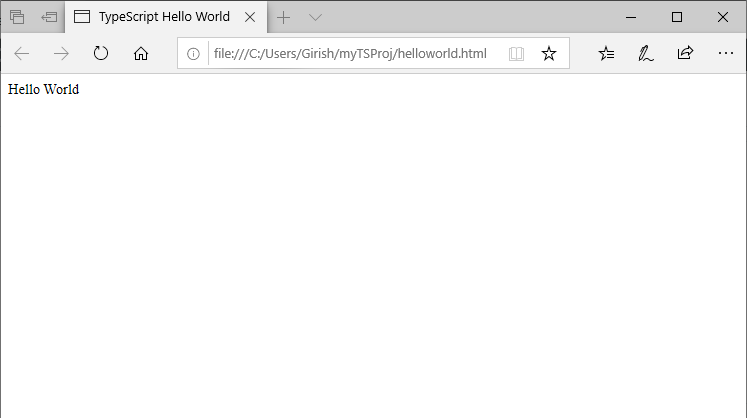
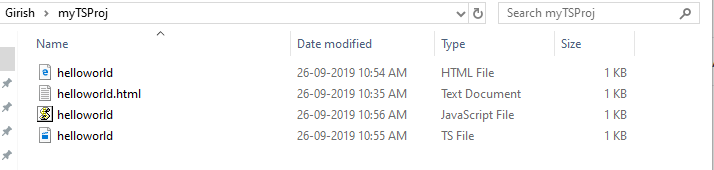
References
- https://www.typescriptlang.org/docs/handbook/typescript-in-5-minutes.html
Learn more about TypeScript language examples in my upcoming TypeScript Blog articles.
Happy Learning!